Building Python Microservices in a Monorepo: A Comprehensive Guide
Nicolás Pérez
Co-founder & Principal Cloud Engineer
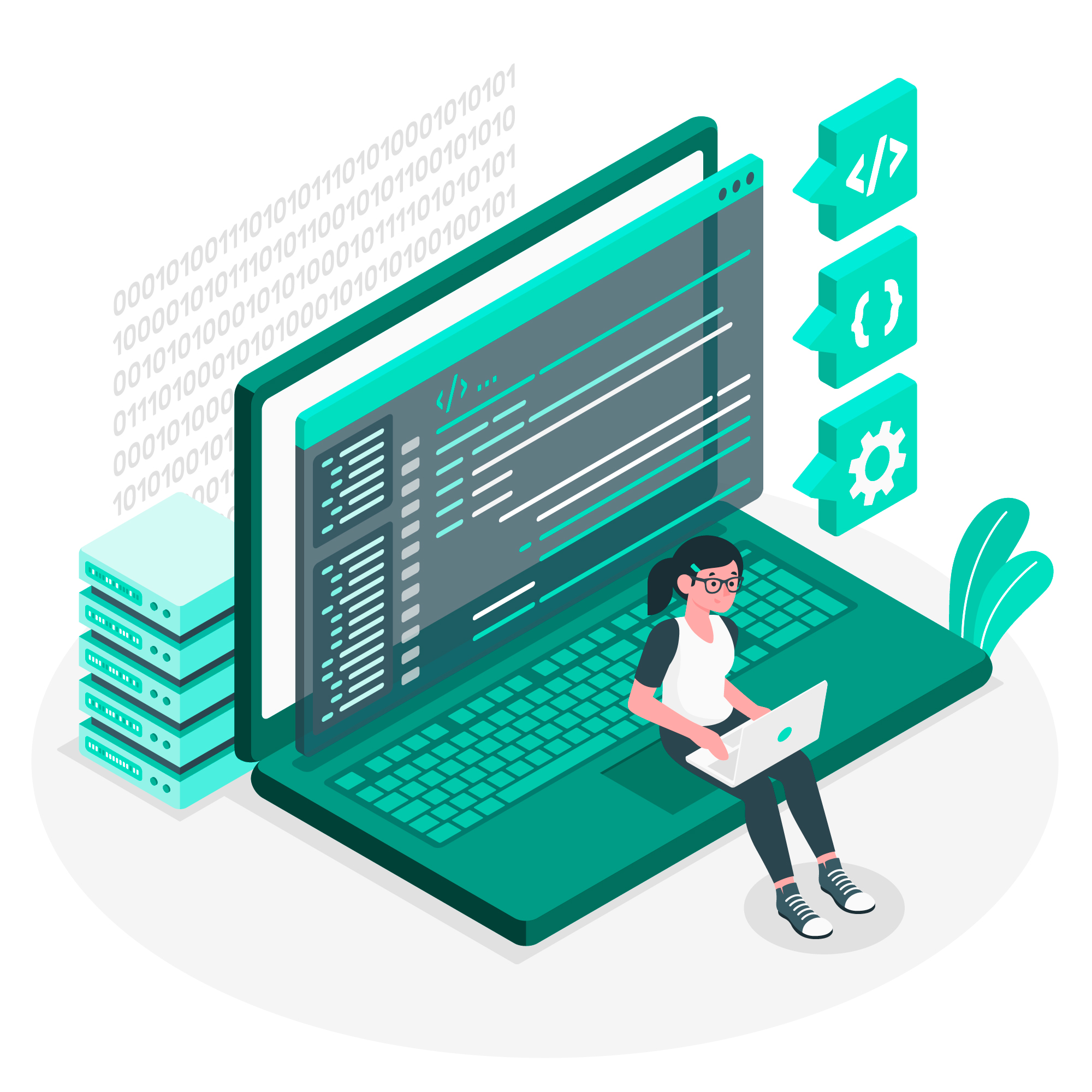
Building Python Microservices in a Monorepo: A Comprehensive Guide
In today's fast-paced software development environment, organizations are constantly looking for ways to streamline their development processes, improve collaboration, and maintain high-quality code. Combining Python microservices with a monorepo approach can offer significant advantages for development teams.
This post walks through a journey implementing Python microservices within a GitHub monorepo, sharing architectural decisions, tools, and patterns that can be established to make this approach successful.
Why Python for Microservices?
Python has emerged as a popular choice for microservice development, and for good reason:
- Developer Productivity: Python's clean syntax and expressive nature dramatically reduce development time, allowing teams to iterate quickly.
- Extensive Ecosystem: The Python ecosystem offers a wealth of libraries and frameworks that can be leveraged for microservice development.
- Versatility: Python's flexibility makes it suitable for a wide range of applications, from data processing to web services.
- Wide Adoption: Python's popularity means it's easier to find experienced developers and community support when facing challenges.
- AI/ML Integration: If your microservices need to incorporate machine learning components, Python's dominance in the ML ecosystem makes integration seamless.
- AI-Assisted Development: Modern AI code assistants are particularly proficient at writing Python code, which can significantly accelerate development and reduce boilerplate. This advantage enables teams to focus on business logic and architecture rather than implementation details.
While Python might not match the raw performance of languages like Rust or Go in all scenarios, the development speed and maintainability advantages often outweigh these considerations for many business applications. It's worth noting that for performance-critical components of your system (such as high-throughput data processing or computationally intensive operations), you might need to implement these specific parts in more performant languages like Rust or Go. The microservices architecture actually facilitates this kind of selective optimization, allowing you to use the right tool for each specific job while keeping the majority of your codebase in Python for productivity and maintainability.
FastAPI: A Modern Framework for Microservices
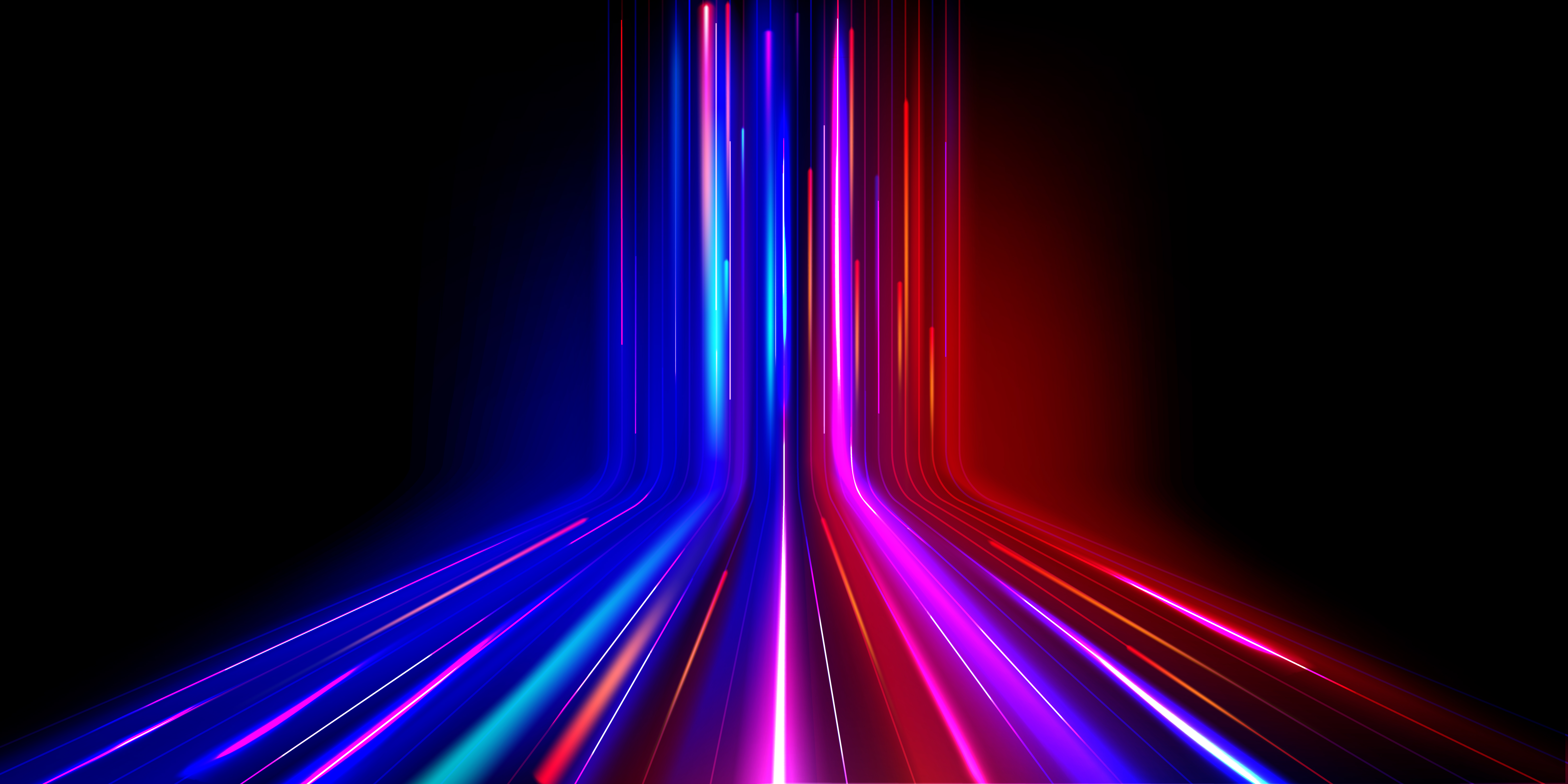
When building Python microservices, the choice of framework significantly impacts development speed, performance, and maintainability. FastAPI has emerged as our framework of choice due to its modern design and impressive feature set.
Native Async Support
FastAPI is built on top of Starlette and Pydantic, leveraging Python's async capabilities:
- High Performance: FastAPI's asynchronous nature means it can handle many concurrent requests efficiently, making it particularly well-suited for I/O-bound operations common in microservices.
- Non-Blocking Operations: Using Python's
async
andawait
syntax, FastAPI enables non-blocking code execution, improving throughput without the complexity of manual thread management. - Concurrent Processing: Services can make multiple external calls concurrently, significantly reducing response times for operations that involve multiple dependencies.
- Careful Implementation Required: While async is powerful for I/O-bound operations, implementation must be careful to avoid blocking the application loop with CPU-intensive code. For computationally heavy tasks, consider using background workers, thread pools, or dedicated microservices to ensure the main application remains responsive.
Pydantic: Type Safety and Validation
Pydantic brings several crucial benefits to microservice development:
- Automatic Validation: Pydantic validates incoming data against declared models, catching issues early and reducing boilerplate code.
- Type Annotations: By leveraging Python's type hints, Pydantic provides better IDE support, documentation, and runtime type checking.
- Schema Generation: Pydantic models automatically generate JSON Schema definitions, which FastAPI uses to create OpenAPI documentation.
Rust Bindings for Performance
A particularly notable feature of Pydantic v2 is its use of Rust bindings:
- Performance Boost: The Rust-based validator is significantly faster than pure Python implementations, especially for complex validation operations.
- Resource Efficiency: The Rust implementation reduces memory usage, which is critical for services handling high volumes of requests.
- Schema Evolution: The performance improvements make it practical to use more complex validation schemes, enabling more robust API contracts.
This combination of async capabilities and strong typing makes FastAPI particularly well-suited for microservice architectures where both performance and maintainability are crucial.
Microservice Architecture: Design Principles
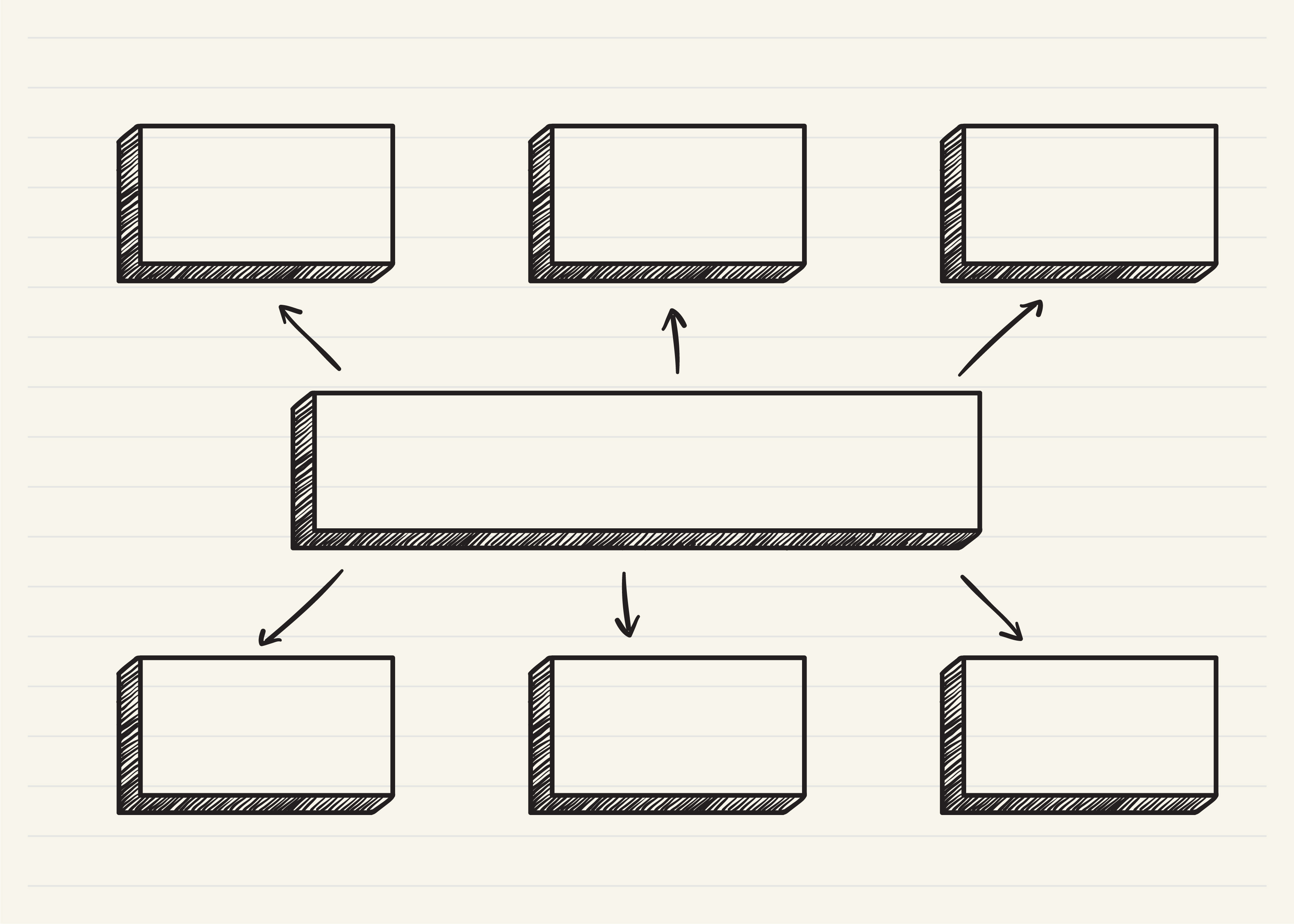
A well-designed microservice architecture follows several key design principles that enhance scalability, maintainability, and deployment flexibility.
Why Microservices?
Before diving into implementation details, it's worth revisiting why a microservice architecture can be a good choice:
- Independent Scaling: Services can be scaled based on their specific resource needs rather than scaling the entire application.
- Technology Flexibility: Different services can use different technologies when appropriate, though we maintain Python as our primary language for consistency.
- Team Autonomy: Teams can develop, test, and deploy services independently, reducing coordination overhead.
- Isolation and Resilience: Failures in one service don't necessarily affect others, improving overall system reliability.
- Targeted Deployments: Updates can be deployed to specific services without rebuilding the entire application.
Core/Internal Microservices
The heart of this architecture consists of core microservices that implement specific business domains:
- Domain Boundaries: Each microservice is responsible for a well-defined domain or capability, following Domain-Driven Design principles.
- Data Ownership: Services own their data and expose it via well-defined APIs rather than sharing databases.
- Autonomous Operation: Core services can function independently, with clearly defined contracts for interaction with other services.
API Gateways and Service Composition
To simplify client interactions and implement cross-cutting concerns, API gateways can be employed:
- Unified Entry Point: Gateways provide a single entry point for clients, abstracting the underlying service architecture.
- Service Composition: For operations requiring data from multiple services, gateways can compose responses, reducing client-side complexity.
- Protocol Translation: Gateways can translate between different protocols (e.g., HTTP to gRPC) as needed.
- Domain-Specific Interfaces: Gateways can implement platform or industry-specific feature languages on top of core services, making interfaces more familiar to end users.
This last point is particularly valuable in specialized industries. For example, in healthcare, standards like FHIR (Fast Healthcare Interoperability Resources) serve a wide range of use cases but contain complex concepts that many users don't fully understand. A gateway can compose and expose FHIR entities in ways that are more intuitive to specific user groups like clinicians or administrators, while the core services maintain the standard implementation.
Communication Patterns
In a microservices architecture, communication patterns are critical for system resilience, scalability, and maintainability. Two primary communication flows can be implemented:
Vertical Communication
Vertical communication flows from API gateways down to core services:
- Synchronous Protocols: Typically REST/HTTP or gRPC for request-response interactions
- Gateway to Service: Client requests come through gateways, which then communicate with appropriate core services
- Aggregation and Transformation: Gateways may call multiple services, aggregate results, and transform responses to match client expectations
Horizontal Communication
Horizontal communication occurs between core services:
- Event-Driven: Services communicate through events published to message brokers (like Kafka, RabbitMQ, or AWS SNS/SQS)
- Asynchronous Processing: Services react to events without direct coupling to event producers
- Domain Events: Services publish events when important domain state changes occur
- Eventual Consistency: This pattern allows for eventual consistency across services, reducing the need for distributed transactions
- Resilience: Event-based communication improves system resilience by reducing direct dependencies between services
The combination of synchronous vertical communication and asynchronous horizontal communication creates a system that balances client responsiveness with internal decoupling and scalability.
Event-Driven Architecture with Kafka
A critical component of horizontal communication strategy can be Apache Kafka, which serves as the backbone of an event-driven architecture.
Why Kafka for Microservices
Kafka provides several advantages for microservice communication:
- High Throughput: Kafka can handle millions of events per second, making it suitable for high-volume systems
- Durability: Events are persisted, allowing services to replay events if needed and recover from failures
- Decoupling: Services only need to know about the event structure, not about other services
- Scalability: Both publishers and consumers can scale independently to handle varying loads
- Stream Processing: Enables real-time analytics and complex event processing
Event Design Principles
When designing events for microservice communication, these principles can be followed:
- Event Ownership: Each service owns specific event types related to its domain
- Schema Evolution: Event schemas evolve carefully to maintain backward compatibility
- Event Enrichment: Events contain sufficient data to minimize the need for additional service calls
- Idempotent Consumers: Services consuming events implement idempotent processing to handle potential duplicates
- Dead Letter Queues: Failed event processing is captured for analysis and potential reprocessing
This event-driven approach can significantly enhance the resilience and scalability of a microservice architecture, enabling truly decoupled services that can evolve independently while maintaining system integrity.
Authentication and Authorization
A crucial aspect of microservice architecture is secure service-to-service communication:
User Authentication
For end-user authentication, JWT tokens can be implemented:
- Centralized Auth Service: A dedicated authentication service issues and validates user tokens.
- Stateless Verification: Services can verify tokens without making network calls to the auth service.
- Claim-Based Authorization: User permissions are encoded in token claims, allowing services to make authorization decisions.
Service-to-Service Authentication
For internal service communication, system tokens can be employed:
- Service Identity: Each service has its own identity and credentials.
- Limited Scope: System tokens have precisely defined scopes that limit what the service can access.
- Short Lifespan: System tokens typically have shorter lifespans than user tokens, reducing the risk from token exposure.
This layered authentication approach ensures that both user requests and inter-service communications are properly authenticated and authorized.
Monorepo Approach: Organizing Your Codebase
A monorepo (monolithic repository) stores all your project's code in a single version control repository, as opposed to using multiple repositories. For microservice architecture, the monorepo approach offers several advantages.
Why Choose a Monorepo?
A monorepo structure for Python microservices can be adopted for several compelling reasons:
Trunk-Based Development
Monorepos facilitate trunk-based development, where teams work collaboratively on a single main branch:
- Continuous Integration: Changes are integrated frequently, reducing merge conflicts and integration issues.
- Smaller, Incremental Changes: Encourages smaller, more focused commits rather than large, infrequent merges.
- Visibility: All code changes are visible to the entire team, fostering collaboration and code review.
Key Benefits
The monorepo approach offers several significant advantages:
- Single Source of Truth: All code is in one place, making it easier to understand the entire system.
- Atomic Changes: You can make changes across multiple services in a single commit, ensuring consistency.
- Simplified Dependency Management: Shared libraries and utilities can be updated in one place.
- Cross-Service Refactoring: Refactoring that spans multiple services is much simpler when all code is in one repository.
- Standardized Tooling: Consistent tooling, testing frameworks, and CI/CD pipelines can be applied across all services.
Reusable CI/CD Pipelines
One of the most significant advantages of a monorepo approach is the ability to create reusable CI/CD workflows:
- Dynamic Workflow Detection: GitHub Actions workflows can detect which projects were affected by changes.
- Targeted Building and Testing: Only affected services are built and tested, saving CI/CD resources.
This approach ensures CI/CD processes run only for services affected by changes, significantly reducing build times and resource usage.
How to Manage a Monorepo Effectively
Managing a monorepo with multiple microservices requires establishing clear patterns and practices:
Established Patterns
Several patterns can be implemented to simplify monorepo usage:
Project Structure
Each project (microservice) follows a consistent structure:
- Each project has a
build.sh
script that:- Installs dependencies
- Runs tests
- Builds artifacts (if needed)
- Each project has a
Dockerfile
for containerization - Shared libraries are kept in a
libs
orcommon
directory
Standardized Scripts
To maintain consistency, standardized scripts across projects can be used:
- build.sh: Handles dependency installation, testing, and building
- run.sh: Sets up any prerequisites and runs the service
- test.sh: Runs tests with appropriate flags and coverage reports
Language Consistency
While microservices allow for technology diversity, maintaining consistency reduces complexity:
- Python as the primary language for microservices
- Consistent framework usage (FastAPI)
- Standardized database access patterns
Local Development
For local development, Docker Compose can run multiple services together, allowing developers to test interactions between services without complex setup.
Deployment Strategies
With a monorepo containing multiple microservices, deployment becomes a critical consideration. Two main deployment approaches can be implemented.
Cloud-Native Deployment (AWS)
For cloud-native deployments, AWS services can be leveraged:
- Load Balancers: Application Load Balancers route traffic to the appropriate services.
- ECS (Elastic Container Service): Container orchestration for running microservices at scale.
- Lambda: Serverless functions for event-driven microservices or infrequently used endpoints.
- Databases: RDS for relational data and DynamoDB for NoSQL requirements.
A typical deployment pipeline might look like:
- Changes are pushed to the monorepo
- CI/CD detects affected services
- Services are built and tested
- Docker images are pushed to ECR (Elastic Container Registry)
- ECS task definitions are updated
- New containers are deployed with blue/green deployment
Kubernetes Deployment
For more flexible deployment options, Kubernetes can be used:
- Helm Charts: Each microservice has its own Helm chart for deployment configuration.
- Containerization: All services are containerized using Docker.
- Deployment Options:
- Cloud-Based: Deploy to managed Kubernetes services like EKS (Amazon Elastic Kubernetes Service)
- On-Premises: Deploy to self-managed Kubernetes clusters in your own data centers
A standard Kubernetes deployment process might include:
- Build Docker images for affected services
- Push images to a container registry
- Update Helm charts with new image versions
- Deploy using Helm, with appropriate rollout strategies
Conclusion
Combining Python microservices with a monorepo approach can be transformative for the development process. The speed and expressiveness of Python, especially with FastAPI, allows teams to develop and iterate quickly. Meanwhile, the monorepo structure ensures consistency across services and simplifies cross-service changes.
The patterns and practices described—standardized project structures, reusable CI/CD workflows, and flexible deployment options—can help maintain high development velocity while ensuring code quality and system reliability.
While this approach might not fit every organization, it can strike an excellent balance between developer productivity, system scalability, and operational efficiency for many use cases.